Leveraging the Entitlement API with AutoLISP & .NET Hybrid
How to invoke Autodesk’s Entitlement API from AutoLISP scripts and integrate with a C#/.NET backend for seamless licensing workflows.

AutoLISP can call external .NET methods, allowing you to embed Entitlement API checks directly within Lisp routines. In this post, we’ll create a hybrid workflow: an AutoLISP command invokes a C# helper to validate and activate licenses via the Entitlement API.
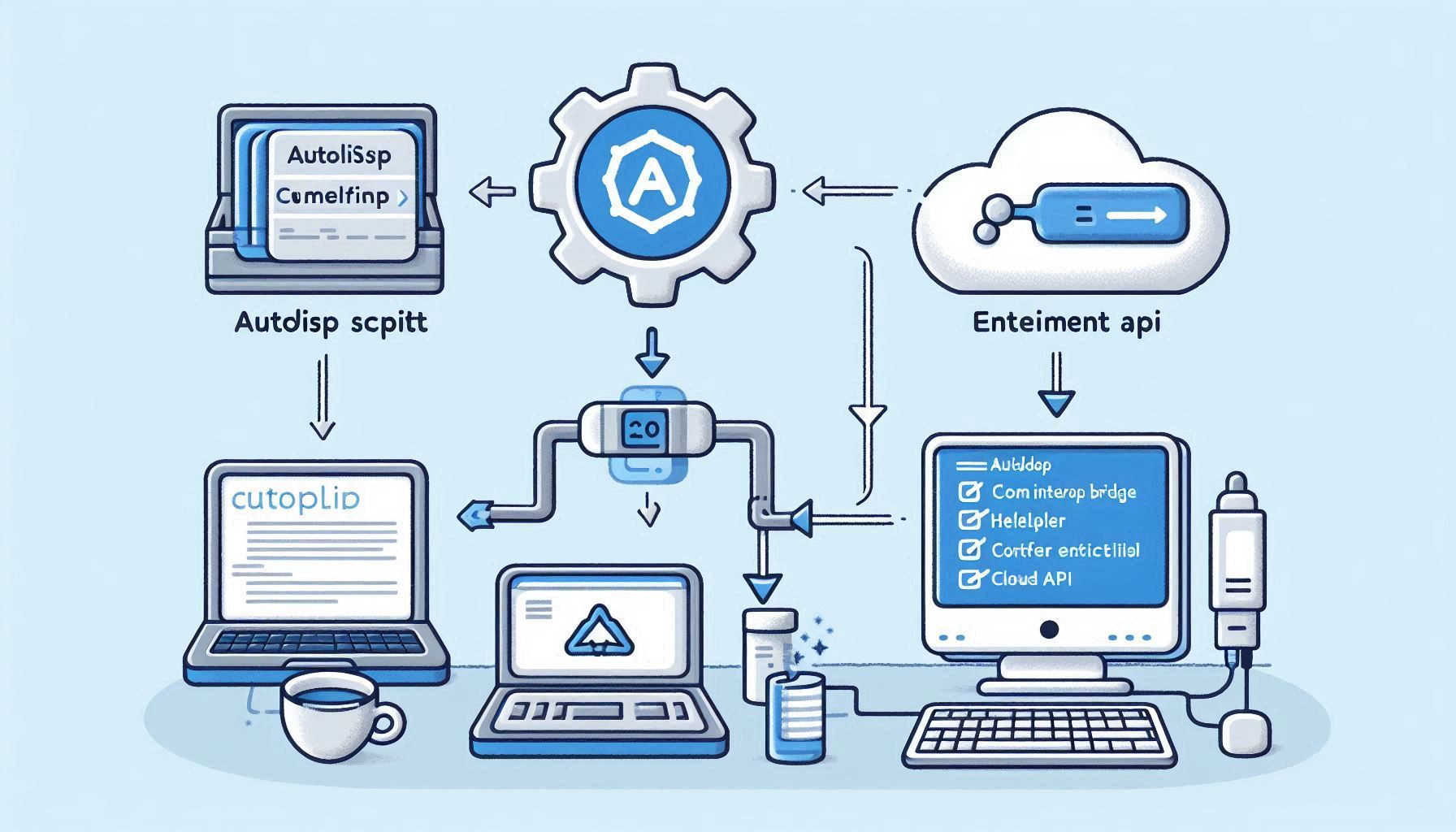
1. Exposing .NET Helper via COM
In your C# class library, mark a helper class COM-visible:
using System.Runtime.InteropServices;
using Autodesk.Entitlement;
[ComVisible(true)]
[Guid("D4A2E5B1-3C7A-4F6E-8F1A-9B2C3D4E5F60")]
public class EntitlementHelper
{
private readonly EntitlementApiClient _client;
public EntitlementHelper()
{
_client = new EntitlementApiClient(
"https://api.autodesk.com/entitlement/v1",
Environment.GetEnvironmentVariable("ENTITLEMENT_API_KEY")
);
}
public bool Validate(string token)
{
var resp = _client.ValidateTokenAsync(token).GetAwaiter().GetResult();
return resp.IsValid;
}
public bool Activate(string token)
{
var machine = Environment.MachineName;
var act = _client.ActivateAsync(token, machine).GetAwaiter().GetResult();
return act.Success;
}
}
Register the assembly for COM interop:
regasm /codebase EntitlementHelper.dll /tlb:EntitlementHelper.tlb
2. Calling from AutoLISP
Load the type library and call the COM methods:
(vl-load-com)
(setq helper (vlax-create-object "EntitlementHelper"))
(setq token (read-line (open "license.token" "r")))
(if (and
(helper.Validate token)
(not (helper.Activate token))
)
(princ "Warning: license validated but activation failed.")
(princ "License validation failed. Plugin unavailable."))
This Lisp routine:
- Loads COM.
- Reads your token file.
- Calls
Validate
thenActivate
, printing errors.
3. Workflow Tips
- Token Caching: Store the last valid token in memory to avoid redundant calls.
- Error Reporting: Use
princ
or dialog boxes to inform users of license issues. - Offline Mode: Fallback to cached validation results when offline.
Next Steps
In Post #5, we’ll cover native ObjectARX integration using C++ and best practices for memory management.
Introduction to Autodesk Entitlement API & Plugin Licensing
A high-level overview of the Entitlement API, its role in plugin licensing, and key concepts for managing licenses in AutoCAD.
Using the Entitlement API in ObjectARX with C++
A practical guide to integrating Autodesk’s Entitlement API with native C++ plugins for AutoCAD using ObjectARX.
Leave a Comment